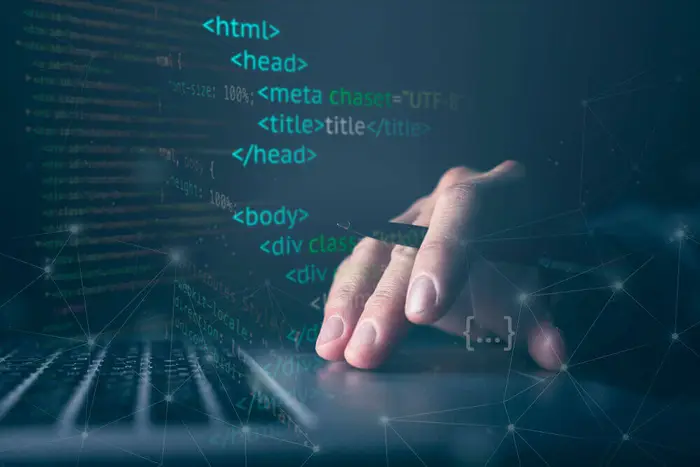
HTML CSS Designs: A Comprehensive Guide
What is HTML?
HTML, or Hypertext Markup Language, is the core language used to structure web content. It forms the backbone of web pages, providing the necessary tags and elements to organize text, images, and other content. When you learn HTML code, you're learning to create the framework of a website. HTML documents are composed of various tags that structure and format the content.
Basic HTML Example
<!DOCTYPE html>
<html>
<head>
<title>HTML CSS Designs Example</title>
</head>
<body>
<h1>Welcome to HTML CSS Designs!</h1>
<p>This is a simple web page structure using HTML.</p>
</body>
</html>
Click to learn more about HTML tagsWhat is CSS?
CSS, or Cascading Style Sheets, defines how HTML elements are presented on a webpage. It controls the layout, colors, fonts, and overall aesthetics. Understanding what CSS defines in HTML is crucial for anyone working with HTML CSS designs. CSS allows you to apply styles consistently across multiple HTML pages, making it an essential tool for web designers.
Basic CSS Example
body {
font-family: Arial, sans-serif;
background-color: #f0f8ff;
}
h1 {
color: #333;
}
p {
color: #555;
font-size: 18px;
}
The Document Object Model (DOM)
The Document Object Model (DOM) is a critical concept in HTML CSS designs. It represents the structure of a web page as a tree of objects. Each HTML tag becomes a node in this tree, allowing scripts to manipulate the content and structure dynamically.
How Browsers Process HTML and CSS
When a browser loads a webpage, it follows these steps to render HTML CSS designs:
- Parsing the HTML: The browser reads the HTML file and creates DOM nodes for each element. For example, <h1> becomes a heading node, and <p> becomes a paragraph node.
- Parsing the CSS: The browser then retrieves and processes the CSS files. It applies the styles to the corresponding DOM nodes, creating the CSS Object Model (CSSOM).
- Building the Render Tree: The DOM and CSSOM are combined to form the render tree, which represents the visual structure of the page.
- Layout: The browser calculates the position and size of each element based on CSS properties like margins, padding, and positioning.
- Painting and Compositing: Finally, the browser paints the pixels on the screen, displaying the styled webpage.
Other Rendering Methods
In addition to the standard rendering process, browsers may use various techniques to improve performance:
- Rendering: This refers to the entire process of turning HTML CSS designs into a visual representation.
- Painting: This involves filling in pixels based on computed styles, including colors, borders, and text.
HTML Tags and Nodes
HTML tags are fundamental to HTML CSS designs. Tags define elements like headings, paragraphs, and links. In the DOM, these tags are also known as nodes. Each tag represents a part of the webpage’s structure.
Common HTML Tags
- <h1> to <h6>: Heading Tags - Define titles or subtitles in descending order of importance.
- <p>: Paragraph Tag - Defines a paragraph of text.
- <a>: Anchor Tag - Creates hyperlinks. It requires an href attribute pointing to a URL.
- <img>: Image Tag - Embeds images. It requires a src attribute pointing to the image file.
- <div>: Division Tag - Groups other HTML elements. Often used for styling purposes.
Basics of CSS and Its Role
CSS is crucial for transforming raw HTML into visually appealing designs. It allows you to control the appearance of web elements, making your pages more engaging and user-friendly. CSS plays several roles in web design:
- Consistency: Ensures a uniform look across multiple pages by centralizing style definitions.
- Reusability: CSS code can be reused across different HTML documents, reducing redundancy.
- Responsiveness: Allows for design adjustments based on screen size, ensuring a good user experience on all devices.
Basic CSS Stylings with Examples
Here are some basic CSS styles with examples:
Text Styling
p {
font-family: 'Arial', sans-serif;
font-size: 16px;
color: #333;
}
Background Color
body {
background-color: #f0f8ff;
}
Margins and Padding
div {
margin: 20px;
padding: 10px;
}
CSS Animations
@keyframes fadeIn {
from { opacity: 0; }
to { opacity: 1; }
}
.fade-in {
animation: fadeIn 2s ease-in;
}
Conclusion
Mastering HTML CSS designs is vital for web developers and designers. From learning HTML code to understanding what CSS defines in HTML, and exploring HTML and CSS animations, this comprehensive guide provides a solid foundation for creating effective web pages. By following the steps outlined, you can ensure your designs are not only functional but also visually appealing and user-friendly.
Whether you're just starting out or looking to refine your skills, understanding HTML CSS designs and how they interact is key to successful web development. Continue exploring and experimenting with these technologies to build dynamic, responsive websites that stand out.